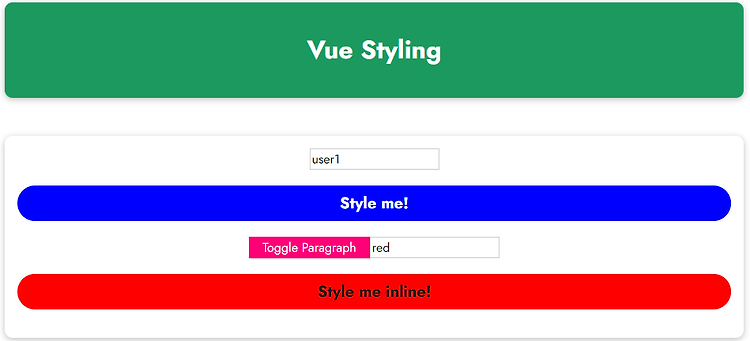
Vue - Dynamic Styling : 구현📘 Frontend/Vue2023. 7. 31. 15:45
Table of Contents
만들어보기
배운것을 활용하여 만들어 볼 페이지는, 사용자로부터 입력을 받아 CSS 클래스와 인라인 스타일을 적용하여 문단을 스타일링하고,
버튼을 클릭하여 문단을 보이거나 숨기는 기능을 구현합니다.
사용자 입력을 CSS 클래스로 적용하는 기능
- 사용자는
<input>
요소를 통해 텍스트를 입력할 수 있습니다. - 이 입력은
v-model
디렉티브를 통해inputClass
데이터 속성과 양방향으로 바인딩됩니다. - 사용자가 입력한 클래스명은 아래의
<p>
요소에 바로 적용됩니다. - 즉, 사용자가 입력한 클래스를
paraClasses
계산된 속성을 통해 Vue에서 적절한 클래스로 연결합니다. - 가능한 클래스는 "user1"과 "user2"입니다.
버튼을 클릭하여 문단을 보이거나 숨기는 기능
- "Toggle Paragraph" 버튼을 클릭하면
toggleParagraphVisibility
메서드가 호출됩니다. - 이 메서드는
paragraphIsVisible
데이터 속성을 반전시켜서 문단의 가시성을 토글합니다. paragraphIsVisible
데이터 속성의 값에 따라 "visible" 또는 "hidden" 클래스가paraClasses
계산된 속성을 통해<p>
요소에 추가됩니다.- 이로 인해 문단이 보이거나 숨겨집니다.
인라인 스타일링 기능
- 사용자는 또 다른
<input>
요소를 통해 배경색을 입력할 수 있습니다. - 이 입력은
v-model
디렉티브를 통해inputBackgroundColor
데이터 속성과 양방향으로 바인딩됩니다. - 사용자가 입력한 배경색은
:style
디렉티브를 사용하여<p>
요소에 인라인 스타일로 적용됩니다. :style
디렉티브의 객체 표기법을 사용하여inputBackgroundColor
데이터 속성을 바탕으로 배경색이 동적으로 변경됩니다.
이러한 기능을 모두 갖춘 Vue 앱은 app.mount('#assignment');
코드를 통해 "assignment" ID를 가진 요소에 마운트되며,
사용자가 입력한 값에 따라 문단의 스타일과 가시성이 동적으로 변경될 수 있습니다.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Vue Basics</title>
<link
href="https://fonts.googleapis.com/css2?family=Jost:wght@400;700&display=swap"
rel="stylesheet"
/>
<link rel="stylesheet" href="styles.css" />
<script src="https://unpkg.com/vue@next" defer></script>
<script src="app.js" defer></script>
</head>
<body>
<header>
<h1>Vue Styling</h1>
</header>
<section id="assignment">
<!-- 1) Fetch the user input and use it as a CSS class -->
<!-- The entered class should be added to the below paragraph -->
<input type="text" v-model="inputClass" />
<!-- (available classes: "user1", "user2") -->
<p
:class="paraClasses"
>
Style me!
</p>
<button @click="toggleParagraphVisibility">Toggle Paragraph</button>
<!-- 2) Use the "visible" and "hidden" classes to show/ hide the above paragraph -->
<!-- Clicking the button should toggle between the two options -->
<!-- 3) Add dynamic inline styling to the below paragraph and let the user enter a background-color -->
<input type="text" v-model="inputBackgroundColor" />
<p :style="{backgroundColor: inputBackgroundColor}">Style me inline!</p>
</section>
</body>
</html>
CSS
.user1 {
background-color: blue;
color: white;
}
.user2 {
background-color: purple;
color: white;
}
.hidden {
display: none;
}
.visible {
display: block;
}
Vue App
const app = Vue.createApp({
data() {
return {
inputClass: '',
paragraphIsVisible: true,
inputBackgroundColor: ''
};
},
computed: {
paraClasses() {
return {
user1: this.inputClass === 'user1',
user2: this.inputClass === 'user2',
visible: this.paragraphIsVisible,
hidden: !this.paragraphIsVisible
};
},
},
methods: {
toggleParagraphVisibility() {
this.paragraphIsVisible = !this.paragraphIsVisible;
},
},
});
app.mount('#assignment');
'📘 Frontend > Vue' 카테고리의 다른 글
Vue - V-for Advanced : Object Looping & Indexing (0) | 2023.07.31 |
---|---|
Vue - Conditional Rendering (0) | 2023.07.31 |
Vue - Dynamic Styling: Class Binding (0) | 2023.07.31 |
Vue - Methods & Computed & Watch & 축약어 정리 (0) | 2023.07.30 |
Vue - Watcher (0) | 2023.07.30 |
@신건우 :: 우주먼지
열심히 살고 싶은 사람의 메모장
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!